
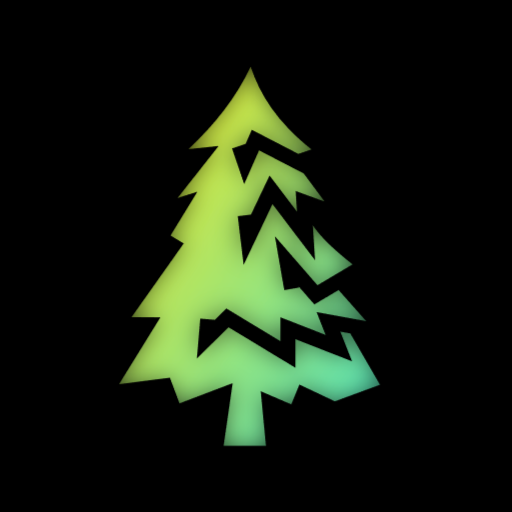
3·
1 year agoPython
Part 1: https://github.com/porotoman99/Advent-of-Code-2023/blob/main/Day 4/part1.py
Part 2: https://github.com/porotoman99/Advent-of-Code-2023/blob/main/Day 4/part2.py
I found out about this event for the first time yesterday and was able to get caught up in time for day 4.
Python
Part 1: https://github.com/porotoman99/Advent-of-Code-2023/blob/main/Day 7/part1.py
Code
import os filePath = os.path.dirname(os.path.realpath(__file__)) inputFilePath = filePath + "\\adventofcode.com_2023_day_7_input.txt" # inputFilePath = filePath + "\\part1.txt" def typeSort(hand): cardCount = { "2": 0, "3": 0, "4": 0, "5": 0, "6": 0, "7": 0, "8": 0, "9": 0, "T": 0, "J": 0, "Q": 0, "K": 0, "A": 0 } for card in hand: cardCount[card] += 1 cardTotals = list(cardCount.values()) cardTotals.sort(reverse=True) if(cardTotals[0] == 5): return 6 elif(cardTotals[0] == 4): return 5 elif(cardTotals[0] == 3 and cardTotals[1] == 2): return 4 elif(cardTotals[0] == 3): return 3 elif(cardTotals[0] == 2 and cardTotals[1] == 2): return 2 elif(cardTotals[0] == 2): return 1 else: return 0 def bucketSort(camelCard): totalScore = 0 cardOrder = ["2","3","4","5","6","7","8","9","T","J","Q","K","A"] hand = camelCard[0] totalScore += cardOrder.index(hand[4]) * 15 ** 1 totalScore += cardOrder.index(hand[3]) * 15 ** 2 totalScore += cardOrder.index(hand[2]) * 15 ** 3 totalScore += cardOrder.index(hand[1]) * 15 ** 4 totalScore += cardOrder.index(hand[0]) * 15 ** 5 return totalScore hands = [] bids = [] with open(inputFilePath) as inputFile: for line in inputFile: lineSplit = line.split() hand = lineSplit[0] bid = lineSplit[1] hands.append(hand) bids.append(bid) bids = [int(bid) for bid in bids] camelCards = list(zip(hands,bids)) typeBuckets = [[],[],[],[],[],[],[]] for camelCard in camelCards: hand = camelCard[0] typeScore = typeSort(hand) typeBuckets[typeScore].append(camelCard) finalCardSort = [] for bucket in typeBuckets: if(len(bucket) > 1): bucket.sort(key=bucketSort) for camelCard in bucket: finalCardSort.append(camelCard) camelScores = [] for camelIndex in range(len(finalCardSort)): scoreMultiplier = camelIndex + 1 camelCard = finalCardSort[camelIndex] camelScores.append(camelCard[1] * scoreMultiplier) print(sum(camelScores))
Part 2: https://github.com/porotoman99/Advent-of-Code-2023/blob/main/Day 7/part2.py
Code
import os filePath = os.path.dirname(os.path.realpath(__file__)) inputFilePath = filePath + "\\adventofcode.com_2023_day_7_input.txt" # inputFilePath = filePath + "\\part1.txt" def typeSort(hand): cardCount = { "J": 0, "2": 0, "3": 0, "4": 0, "5": 0, "6": 0, "7": 0, "8": 0, "9": 0, "T": 0, "Q": 0, "K": 0, "A": 0 } for card in hand: cardCount[card] += 1 jokerCount = cardCount["J"] cardCount["J"] = 0 cardTotals = list(cardCount.values()) cardTotals.sort(reverse=True) if(cardTotals[0] + jokerCount == 5): return 6 elif(cardTotals[0] + jokerCount == 4): return 5 elif( cardTotals[0] + jokerCount == 3 and cardTotals[1] == 2 or cardTotals[0] == 3 and cardTotals[1] + jokerCount == 2 ): return 4 elif(cardTotals[0] + jokerCount == 3): return 3 elif( cardTotals[0] + jokerCount == 2 and cardTotals[1] == 2 or cardTotals[0] == 2 and cardTotals[1] + jokerCount == 2 ): return 2 elif(cardTotals[0] + jokerCount == 2): return 1 else: return 0 def bucketSort(camelCard): totalScore = 0 cardOrder = ["J","2","3","4","5","6","7","8","9","T","Q","K","A"] hand = camelCard[0] totalScore += cardOrder.index(hand[4]) * 15 ** 1 totalScore += cardOrder.index(hand[3]) * 15 ** 2 totalScore += cardOrder.index(hand[2]) * 15 ** 3 totalScore += cardOrder.index(hand[1]) * 15 ** 4 totalScore += cardOrder.index(hand[0]) * 15 ** 5 return totalScore hands = [] bids = [] with open(inputFilePath) as inputFile: for line in inputFile: lineSplit = line.split() hand = lineSplit[0] bid = lineSplit[1] hands.append(hand) bids.append(bid) bids = [int(bid) for bid in bids] camelCards = list(zip(hands,bids)) typeBuckets = [[],[],[],[],[],[],[]] for camelCard in camelCards: hand = camelCard[0] typeScore = typeSort(hand) typeBuckets[typeScore].append(camelCard) finalCardSort = [] for bucket in typeBuckets: if(len(bucket) > 1): bucket.sort(key=bucketSort) for camelCard in bucket: finalCardSort.append(camelCard) camelScores = [] for camelIndex in range(len(finalCardSort)): scoreMultiplier = camelIndex + 1 camelCard = finalCardSort[camelIndex] camelScores.append(camelCard[1] * scoreMultiplier) print(sum(camelScores))
I tried to do this one as quickly as possible, so the code is more messy than I would prefer, but it works, and I don’t think the solution is too bad overall.
Edit: I went back and changed it to be a bit better. Here are my new solutions:
Part 1 v2: https://github.com/porotoman99/Advent-of-Code-2023/blob/main/Day 7/part1v2.py
Code
import os filePath = os.path.dirname(os.path.realpath(__file__)) inputFilePath = filePath + "\\adventofcode.com_2023_day_7_input.txt" # inputFilePath = filePath + "\\part1.txt" CARD_ORDER = "23456789TJQKA" def typeSort(camelCard): cardCount = {} for card in CARD_ORDER: cardCount[card] = 0 hand = camelCard[0] for card in hand: cardCount[card] += 1 cardTotals = list(cardCount.values()) cardTotals.sort(reverse=True) if(cardTotals[0] == 5): return 6 elif(cardTotals[0] == 4): return 5 elif(cardTotals[0] == 3 and cardTotals[1] == 2): return 4 elif(cardTotals[0] == 3): return 3 elif(cardTotals[0] == 2 and cardTotals[1] == 2): return 2 elif(cardTotals[0] == 2): return 1 else: return 0 def handSort(camelCard): totalScore = 0 hand = camelCard[0] totalScore += CARD_ORDER.index(hand[4]) * 15 ** 1 totalScore += CARD_ORDER.index(hand[3]) * 15 ** 2 totalScore += CARD_ORDER.index(hand[2]) * 15 ** 3 totalScore += CARD_ORDER.index(hand[1]) * 15 ** 4 totalScore += CARD_ORDER.index(hand[0]) * 15 ** 5 return totalScore hands = [] bids = [] with open(inputFilePath) as inputFile: for line in inputFile: lineSplit = line.split() hand = lineSplit[0] bid = lineSplit[1] hands.append(hand) bids.append(int(bid)) camelCards = list(zip(hands,bids)) camelCards = sorted(camelCards, key=lambda x: (typeSort(x), handSort(x))) camelScores = [] for camelIndex in range(len(camelCards)): scoreMultiplier = camelIndex + 1 camelCard = camelCards[camelIndex] camelScores.append(camelCard[1] * scoreMultiplier) print(sum(camelScores))
Part 2 v2: https://github.com/porotoman99/Advent-of-Code-2023/blob/main/Day 7/part2v2.py
Code
import os filePath = os.path.dirname(os.path.realpath(__file__)) inputFilePath = filePath + "\\adventofcode.com_2023_day_7_input.txt" # inputFilePath = filePath + "\\part1.txt" CARD_ORDER = "J23456789TQKA" def typeSort(camelCard): cardCount = {} for card in CARD_ORDER: cardCount[card] = 0 hand = camelCard[0] for card in hand: cardCount[card] += 1 jokerCount = cardCount["J"] cardCount["J"] = 0 cardTotals = list(cardCount.values()) cardTotals.sort(reverse=True) if(cardTotals[0] + jokerCount == 5): return 6 elif(cardTotals[0] + jokerCount == 4): return 5 elif( cardTotals[0] + jokerCount == 3 and cardTotals[1] == 2 or cardTotals[0] == 3 and cardTotals[1] + jokerCount == 2 ): return 4 elif(cardTotals[0] + jokerCount == 3): return 3 elif( cardTotals[0] + jokerCount == 2 and cardTotals[1] == 2 or cardTotals[0] == 2 and cardTotals[1] + jokerCount == 2 ): return 2 elif(cardTotals[0] + jokerCount == 2): return 1 else: return 0 def handSort(camelCard): totalScore = 0 hand = camelCard[0] totalScore += CARD_ORDER.index(hand[4]) * 15 ** 1 totalScore += CARD_ORDER.index(hand[3]) * 15 ** 2 totalScore += CARD_ORDER.index(hand[2]) * 15 ** 3 totalScore += CARD_ORDER.index(hand[1]) * 15 ** 4 totalScore += CARD_ORDER.index(hand[0]) * 15 ** 5 return totalScore hands = [] bids = [] with open(inputFilePath) as inputFile: for line in inputFile: lineSplit = line.split() hand = lineSplit[0] bid = lineSplit[1] hands.append(hand) bids.append(int(bid)) camelCards = list(zip(hands,bids)) camelCards = sorted(camelCards, key=lambda x: (typeSort(x), handSort(x))) camelScores = [] for camelIndex in range(len(camelCards)): scoreMultiplier = camelIndex + 1 camelCard = camelCards[camelIndex] camelScores.append(camelCard[1] * scoreMultiplier) print(sum(camelScores))